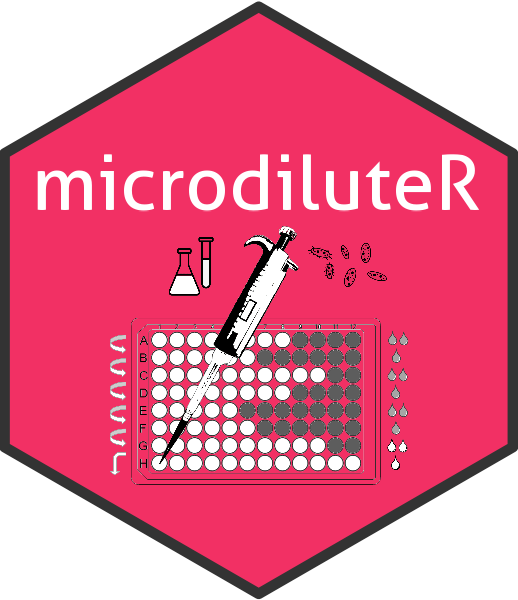
Tidy multiple 96-well plates via parameters
Source:R/tidy_plates_via_params.R
tidy_plates_via_params.Rd
This function processes raw plates data from photometer measurements, adds metadata via user-specified parameter values, and combines processed data into a single data frame.
Arguments
- input_data
Either a folder path containing raw data files or a list of data frames.
- direction
A character vector specifying the orientation of the plate layout. It can be either "horizontal" or "vertical".
- group_IDs
A character vector providing group identifiers for each experiment.
- experiment_names
A character vector providing names for each experiment. The hierarchy is group > experiment, i.e. within a single group, there might be several experiments taking place (e.g. multiple extracts from the same plant species tested with plant species being the group and type of extract being the experiment).
- validity_method
A character vector specifying the method for determining cell validity. It can be either "threshold" (i.e. samples are validated based on a common absorption maximum) or "samples" (i.e. samples are manually specified as invalid).
- threshold
A numeric threshold value. Applied if
validity_method
is set to 'threshold'.- invalid_samples
A character vector containing well positions (e.g. "A-3", "B-8",...) of invalid samples. Applied if
validity_method
is set to 'samples'.- treatment_labels
A character vector containing treatment labels.
- concentration_levels
A numeric vector containing concentration levels.
- ...
Additional arguments to be passed to
read_plates
.
Details
This function processes photometer data from multiple experiments and adds metadata based on user-set parameters if
the experimental layout is repeated across plates and should by synchronized. It supports two methods for
determining cell validity: "threshold" and "invalid". If "threshold" method is chosen, the validity of each cell
is determined based on a specified threshold value. If "sample" method is chosen, samples at specified well positions
on the plate are considered invalid. The function generates lists of treatments and concentration levels based on the
direction parameter, i.e. the direction of the treatments and concentration levels applied (either horizontally or
vertically on the plate). If the plate layout and, thus, the metadata changes across plates, then function
tidy_plates_via_prompts
might be a better choice since it helps the user to add metadata for each plate separately
based on user prompts. If there is only one plate, where metadata should be added, then tidy_single_plate
should be used.
For all three functions, tidy_single_plate
, tidy_plates_via_params
, and tidy_plates_via_prompts
, to work
properly, file names should provide a file identifier (i.e. "bma" in case there are additional but not relevant files in
the folder), a group identifier (i.e. starting with "grp" followed by an incrementing number), an identifier for
experiments (starting with "exp" followed by a number, e.g. "exp1") and an identifier for timepoints (starting with the
upper- or lower-case letter t followed by an incrementing number, e.g. "T0" or "t0").
Examples
# Load example data
data(bma)
# Add metadata from user parameters
bma_tidy <- tidy_plates_via_params(input_data = bma,
direction = "horizontal",
group_IDs = paste0("Group_", letters[1:2]),
experiment_names = c("Experiment 1", "Experiment 2"),
validity_method = "threshold",
threshold = 1,
treatment_labels = LETTERS[1:8],
concentration_levels = seq(from=80, to=10, length.out=8))
bma_tidy # View tidy data
#> # A tibble: 384 × 9
#> Position Value Validity Treatment Concentration Timepoint File Group
#> <chr> <dbl> <chr> <chr> <dbl> <chr> <chr> <chr>
#> 1 A-1 0.342 valid A 80 T0 bma_grp1_exp… Grou…
#> 2 A-2 0.354 valid A 80 T0 bma_grp1_exp… Grou…
#> 3 A-3 0.36 valid A 80 T0 bma_grp1_exp… Grou…
#> 4 A-4 0.36 valid A 80 T0 bma_grp1_exp… Grou…
#> 5 A-5 0.352 valid A 80 T0 bma_grp1_exp… Grou…
#> 6 A-6 0.363 valid A 80 T0 bma_grp1_exp… Grou…
#> 7 A-7 0.361 valid A 80 T0 bma_grp1_exp… Grou…
#> 8 A-8 0.352 valid A 80 T0 bma_grp1_exp… Grou…
#> 9 A-9 0.356 valid A 80 T0 bma_grp1_exp… Grou…
#> 10 A-10 0.351 valid A 80 T0 bma_grp1_exp… Grou…
#> # ℹ 374 more rows
#> # ℹ 1 more variable: Experiment <chr>