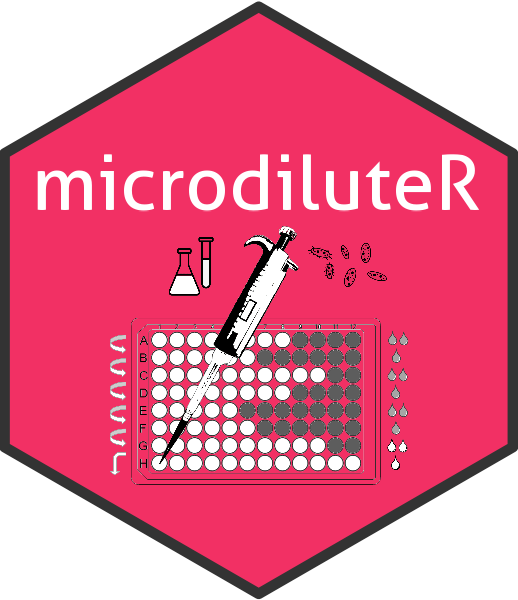
Calculate and visualize growth performance
Source:R/growth_performance.R
calculate_growth_performance.Rd
calculate_growth_performance
standardizes data by subtracting the average value of the control group from each treatment level
for each concentration level, applied within each experiment. It assumes the input data is a data frame with columns 'Experiment', 'Concentration', 'Treatment',
and 'Value', where 'Concentration' represents different concentration levels, 'Treatment' represents different
treatment groups, and 'Value' represents the corresponding absorption values.
calculate_percentage_change
calculates the percentage change between a vector of values (or a
single value) and a reference value as the baseline. If a value in the vector is less than the reference, it returns
the negative percentage difference; otherwise, it returns the positive percentage difference.
summarize_growth_performance
summarizes a data frame containing growth performance by
computing the mean and either the standard error or standard deviation.
plot_growth_performance
visualizes growth performance using bar charts with error bars.
Usage
calculate_growth_performance(
input_data,
treatment_grouping = FALSE,
concentration_grouping = FALSE,
group = "Group",
experiment = "Experiment",
treatment = "Treatment",
concentration = "Concentration",
timepoint = "Timepoint",
value = "Value",
control_mean = "control_mean"
)
calculate_percentage_change(input, reference)
summarize_growth_performance(
input_data,
compute_sd = FALSE,
grouping = c("Group", "Treatment", "Concentration", "Timepoint"),
treatment = "Treatment",
value = "Value"
)
plot_growth_performance(
input_data,
stats_data = NULL,
level_unit = NULL,
treatment_order = NULL,
apply_sign_test = FALSE,
grouping = NULL,
x_var = "Treatment",
y_var = "mean",
error_var = "stderr",
x_lab = "Treatment",
y_lab = NULL,
fill_var = "Concentration",
row_facets = NULL,
col_facets = "Group",
value = "Value",
p_values = "p.signif",
level_colors = NULL,
...
)
Arguments
- input_data
A data frame containing summarized data, e.g. from function call to
summarize_growth_performance
.- treatment_grouping
A Boolean value that specifies whether or not (default) there is a treatment grouping within the plate.
- concentration_grouping
A Boolean value that specifies whether or not (default) there is a concentration grouping within the plate.
- group
The column containing group information. Defaults to 'Group'.
- experiment
The column containing experiment information. Defaults to 'Experiment'. The hierarchy is group > experiment, i.e. within a single group, there might be several experiments taking place (e.g. multiple extracts from the same plant species tested with plant species being the group and type of extract being the experiment).
- treatment
The column containing treatment information. Defaults to 'Treatment'.
- concentration
The column containing concentration information. Defaults to 'Concentration'.
- timepoint
The column containing timepoint information. Defaults to 'Timepoint'.
- value
The column containing the absorption values to be assessed via
apply_sign_test
. Defaults to 'Value'.- control_mean
he column containing the absorption values to calculate growth performance. Defaults to 'control_mean'.
- input
A single numeric value.
- reference
A single numeric value serving as the baseline for comparison.
- compute_sd
Logical, indicating whether to compute the standard deviation (default) or standard error.
- grouping
Optional. A character vector specifying the grouping variables on which to apply the sign test. If not specified and 'apply_sign_test' is set to TRUE, then the test will be applied on the whole dataset.
- stats_data
Optional. A data frame containing growth performance data, e.g. from function call to
calculate_growth_performance
. Only necessary, if 'apply_sign_test' parameter is set to TRUE.- level_unit
Optional. The unit of applied concentrations to display on the y-axis.
- treatment_order
Optional. An alternative order of factor levels on the x-axis.
- apply_sign_test
Logical. Should the sign test be applied to specified levels? For this, the 'stats_data' and 'grouping' parameters need to be specified.
- x_var
The variable name for the x-axis. Defaults to "Treatment".
- y_var
The variable name for the y-axis. Defaults to "mean".
- error_var
The variable name to generate the error bars. Defaults to 'stderr'.
- x_lab
The label for the x-axis. Defaults to "Treatment".
- y_lab
Optional. The label for the y-axis. If not provided will return "Relative growth performance".
- fill_var
The variable used to fill facets. Defaults to "Concentration".
- row_facets
A character vector specifying nested column facets. Defaults to
NULL
.- col_facets
A character vector specifying nested row facets. Defaults to "Group".
- p_values
The column containing the (adjusted) p-values. Defaults to 'p.adj.signif' from a function call to
apply_sign_test
andrstatix::sign_test
.- level_colors
Optional. The colors for different levels. If not specified, will be determined based on levels of 'fill_var' using
gray.colors
.- ...
Additional arguments to be passed to
apply_sign_test
.
Value
calculate_growth_performance
returns a modified data frame with the control mean subtracted from each treatment level for each concentration level, applied within each experiment.
calculate_percentage_change
returns a numeric vector containing the percentage change for each value in the vector compared to the reference.
summarize_growth_performance
returns a data frame containing the summary statistics.
plot_growth_performance
returns a ggplot object.